【字符串处理算法】最长连续字符及其出现次数的算法设计及C代码实现
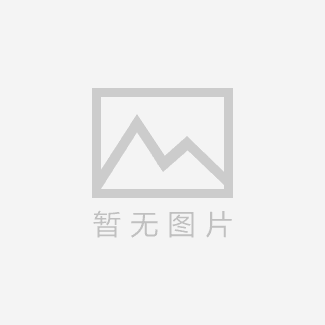
问题描述
给定一个字符串,设计一个算法找出其中最长的连续字符以及它的出现次数。
算法设计
为了找到最长的连续字符及其出现次数,我们可以采用以下步骤:
- 初始化计数器变量count为0,最长连续字符变量maxLength为0,当前连续字符变量currentLength为0。
- 遍历字符串的每个字符:
- 如果当前字符与前一个字符相同,将当前连续字符变量currentLength递增。
- 如果当前字符与前一个字符不同,将最长连续字符变量maxLength更新为当前连续字符变量currentLength的较大值,并将当前连续字符变量currentLength重置为1。
- 将计数器变量count更新为当前字符的出现次数。
- 将最长连续字符变量maxLength与当前连续字符变量currentLength进行比较,保留较大值。
- 返回最长连续字符及其出现次数。
C代码实现
#include#include void longestConsecutive(char *str, int *maxLength, int *count) { int len = strlen(str); int currentLength = 1; *maxLength = 1; *count = 1; for (int i = 1; i < len; i++) { if (str[i] == str[i-1]) { currentLength++; } else { if (currentLength > *maxLength) { *maxLength = currentLength; } currentLength = 1; } (*count)++; } if (currentLength > *maxLength) { *maxLength = currentLength; } } int main() { char str[100]; int maxLength, count; printf("请输入一个字符串:"); scanf("%s", str); longestConsecutive(str, &maxLength, &count); printf("最长连续字符及其出现次数: "); printf("字符:%c ", str[0]); printf("出现次数:%d ", count); return 0; }