遗传算法的基本概念和实现(附 Java 实现案例)
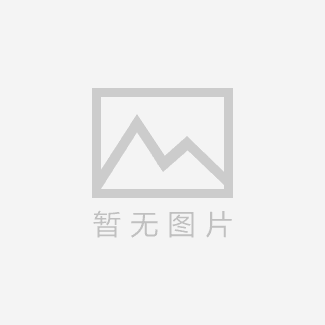
遗传算法的基本概念
遗传算法(Genetic Algorithm,GA)是一种模拟自然界中生物进化过程的优化算法。它通过模拟生物的遗传、突变、适应性等进化过程,通过不断迭代寻找问题的最优解。遗传算法由三个基本操作组成,即选择(Selection)、交叉(Crossover)和变异(Mutation),它们模拟了生物进化中的选择优良个体、基因交换和基因突变的过程。
遗传算法的实现
遗传算法的实现过程可以分为以下几个步骤:
- 初始化种群:随机生成一组初始解作为种群。
- 适应度评估:计算每个个体的适应度,用于度量解的优劣。
- 选择操作:根据适应度选择一定数量的个体作为父代,可以采用轮盘赌选择、排名选择等方法。
- 交叉操作:选取父代个体进行基因交叉,生成子代个体。
- 变异操作:对子代进行基因变异,引入新的基因信息。
- 更新种群:根据选择、交叉和变异得到的子代个体更新种群。
- 迭代优化:重复执行以上步骤,直到达到终止条件。
通过不断迭代,每一代的个体逐渐趋于最优解,从而得到优化问题的解。
遗传算法的 Java 实现案例
以下是一个使用 Java 实现的简单遗传算法案例,用于求解一个简单的函数最优化问题。
import java.util.Random; public class GeneticAlgorithm { private static final int POPULATION_SIZE = 100; private static final int MAX_GENERATIONS = 100; private static final double CROSSOVER_RATE = 0.6; private static final double MUTATION_RATE = 0.02; private static Random random = new Random(); private static double fitnessFunction(double x) { return Math.sin(x) + Math.cos(2 * x); } private static void initializePopulation(double[] population) { for (int i = 0; i < population.length; i++) { population[i] = random.nextDouble() * 10 - 5; } } private static void evaluatePopulation(double[] population, double[] fitness) { for (int i = 0; i < population.length; i++) { fitness[i] = fitnessFunction(population[i]); } } private static double selectParent(double[] population, double[] fitness) { double totalFitness = 0; for (double f : fitness) { totalFitness += f; } double randomFitness = random.nextDouble() * totalFitness; double sum = 0; for (int i = 0; i < population.length; i++) { sum += fitness[i]; if (sum > randomFitness) { return population[i]; } } return population[population.length - 1]; } private static double crossover(double parent1, double parent2) { if (random.nextDouble() < CROSSOVER_RATE) { return (parent1 + parent2) / 2; } else { return parent1; } } private static double mutate(double offspring) { if (random.nextDouble() < MUTATION_RATE) { return offspring + random.nextGaussian(); // Gaussian mutation } else { return offspring; } } public static void main(String[] args) { double[] population = new double[POPULATION_SIZE]; double[] fitness = new double[POPULATION_SIZE]; initializePopulation(population); evaluatePopulation(population, fitness); for (int generation = 0; generation < MAX_GENERATIONS; generation++) { double[] offspring = new double[POPULATION_SIZE]; for (int i = 0; i < POPULATION_SIZE; i++) { double parent1 = selectParent(population, fitness); double parent2 = selectParent(population, fitness); double child = crossover(parent1, parent2); double newChild = mutate(child); offspring[i] = newChild; } population = offspring; evaluatePopulation(population, fitness); } double bestIndividual = population[0]; double bestFitness = fitness[0]; for (int i = 1; i < POPULATION_SIZE; i++) { if (fitness[i] > bestFitness) { bestIndividual = population[i]; bestFitness = fitness[i]; } } System.out.println("Best individual: " + bestIndividual); System.out.println("Best fitness: " + bestFitness); } }
上述代码通过遗传算法求解函数 f(x) = sin(x) + cos(2x) 的最大值。通过初始化种群,适应度评估,选择操作,交叉操作和变异操作的迭代过程,最终得到了最优解。