常见几种加密算法的Python实现
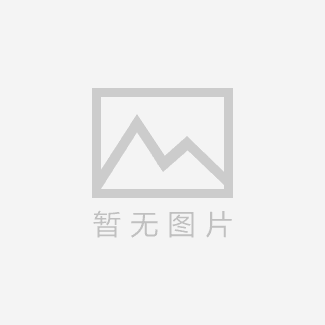
常见几种加密算法的Python实现
加密算法是信息安全领域的重要内容之一。本文将介绍几种常见的加密算法,并提供其在Python中的实现。
对称加密算法
对称加密算法是一种加密和解密使用相同密钥的算法。常见的对称加密算法有DES、3DES和AES。
DES(Data Encryption Standard)是一种对称加密算法,由IBM研发并于1977年被美国联邦政府采用。下面是Python中使用DES算法进行加密和解密的示例代码:
from Crypto.Cipher import DES
from Crypto.Util.Padding import pad, unpad
key = b'01234567' # 密钥必须为8个字节
plaintext = b'hello world'
cipher = DES.new(key, DES.MODE_ECB)
ciphertext = cipher.encrypt(pad(plaintext, DES.block_size))
print("加密后的密文:", ciphertext)
decipher = DES.new(key, DES.MODE_ECB)
decrypted_text = unpad(decipher.decrypt(ciphertext), DES.block_size)
print("解密后的明文:", decrypted_text)
3DES(Triple Data Encryption Standard)是基于DES的对称加密算法,采用了3个不同的密钥。下面是Python中使用3DES算法进行加密和解密的示例代码:
from Crypto.Cipher import DES3
from Crypto.Util.Padding import pad, unpad
key = b'0123456789abcdef01234567' # 密钥必须为24个字节
plaintext = b'hello world'
cipher = DES3.new(key, DES3.MODE_ECB)
ciphertext = cipher.encrypt(pad(plaintext, DES3.block_size))
print("加密后的密文:", ciphertext)
decipher = DES3.new(key, DES3.MODE_ECB)
decrypted_text = unpad(decipher.decrypt(ciphertext), DES3.block_size)
print("解密后的明文:", decrypted_text)
AES(Advanced Encryption Standard)是一种高级的对称加密算法,它使用128、192或256比特的密钥对数据进行加密和解密。下面是Python中使用AES算法进行加密和解密的示例代码:
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
key = b'0123456789abcdef0123456789abcdef' # 密钥必须为16、24或32个字节
plaintext = b'hello world'
cipher = AES.new(key, AES.MODE_ECB)
ciphertext = cipher.encrypt(pad(plaintext, AES.block_size))
print("加密后的密文:", ciphertext)
decipher = AES.new(key, AES.MODE_ECB)
decrypted_text = unpad(decipher.decrypt(ciphertext), AES.block_size)
print("解密后的明文:", decrypted_text)
非对称加密算法
非对称加密算法是一种使用不同的密钥进行加密和解密的算法。常见的非对称加密算法有RSA和ECC。
RSA(Rivest, Shamir和Adleman)是一种基于大数分解的非对称加密算法。下面是Python中使用RSA算法进行加密和解密的示例代码:
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_v1_5
from Crypto.Util.Padding import pad, unpad
plaintext = b'hello world'
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
cipher = PKCS1_v1_5.new(RSA.import_key(public_key))
ciphertext = cipher.encrypt(pad(plaintext,16))
print("加密后的密文:", ciphertext)
decipher = PKCS1_v1_5.new(RSA.import_key(private_key))
decrypted_text = unpad(decipher.decrypt(ciphertext), 16)
print("解密后的明文:", decrypted_text)
ECC(Elliptic Curve Cryptography)是一种基于椭圆曲线的非对称加密算法。下面是Python中使用ECC算法进行加密和解密的示例代码:
from cryptography.hazmat.primitives.asymmetric import ec
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.serialization import Encoding, PublicFormat
plaintext = b'hello world'
private_key = ec.generate_private_key(ec.SECP256R1())
public_key = private_key.public_key().public_bytes(Encoding.PEM, PublicFormat.SubjectPublicKeyInfo)
ciphertext = private_key.exchange(ec.ECDH(), ec.EllipticCurvePublicKey.from_encoded_point(ec.SECP256R1(), public_key)).hex()
print("加密后的密文:", ciphertext)
哈希函数
哈希函数是一种将数据转换为固定长度的唯一字符串的算法。常见的哈希函数有MD5和SHA系列(SHA-1、SHA-224、SHA-256、SHA-384、SHA-512等)。
MD5(Message Digest Algorithm 5)是一种常用的哈希函数,它将任意长度的数据转换为128比特的字符串。下面是Python中使用MD5算法进行哈希的示例代码:
import hashlib
plaintext = b'hello world'
md5_hash = hashlib.md5()
md5_hash.update(plaintext)
hash_value = md5_hash.hexdigest()
print("MD5哈希值:", hash_value)
SHA(Secure Hash Algorithm)系列是一组哈希函数,用于将数据转换为不同长度的哈希值。下面是Python中使用SHA-256算法进行哈希的示例代码:
import hashlib
plaintext = b'hello world'
sha256_hash = hashlib.sha256()
sha256_hash.update(plaintext)
hash_value = sha256_hash.hexdigest()
print("SHA-256哈希值:", hash_value)
以上是几种常见的加密算法在Python中的实现示例。根据具体的需求和应用场景,选择适合的加密算法可以保障数据的安全性。