Go加密解密算法总结
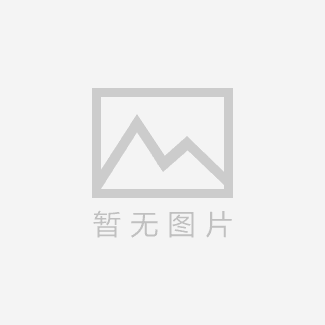
Go加密解密算法总结
Go语言作为一种强大的编程语言,提供了丰富的加密解密算法库,使得在Go中实现数据的保密性和完整性变得更加简单和高效。本文将对几种常见的Go加密解密算法进行总结和介绍,并给出相应的代码示例。
对称加密算法
对称加密算法是指加密和解密使用相同的密钥的算法。Go语言中最常用的对称加密算法有AES(Advanced Encryption Standard)和DES(Data Encryption Standard)。
下面是使用Go语言实现AES加密解密的示例代码:
package main import ( "crypto/aes" "crypto/cipher" "fmt" ) func encrypt(plainText []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } // 填充明文 blockSize := block.BlockSize() plainText = append(plainText, bytes.Repeat([]byte{byte(blockSize - len(plainText)%blockSize)}, blockSize-len(plainText)%blockSize)...) // 加密 cipherText := make([]byte, len(plainText)) mode := cipher.NewCBCEncrypter(block, key[:blockSize]) mode.CryptBlocks(cipherText, plainText) return cipherText, nil } func decrypt(cipherText []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } // 解密 plainText := make([]byte, len(cipherText)) mode := cipher.NewCBCDecrypter(block, key[:blockSize]) mode.CryptBlocks(plainText, cipherText) // 去除填充 plainText = PKCS7UnPadding(plainText) return plainText, nil } func main() { key := []byte("0123456789abcdef") plainText := []byte("Hello, World!") cipherText, err := encrypt(plainText, key) if err != nil { fmt.Println("Error encrypting:", err) return } fmt.Println("Cipher text:", cipherText) decryptedText, err := decrypt(cipherText, key) if err != nil { fmt.Println("Error decrypting:", err) return } fmt.Println("Decrypted text:", decryptedText) }
非对称加密算法
非对称加密算法是指加密和解密使用不同的密钥的算法。Go语言中支持的非对称加密算法有RSA(Rivest-Shamir-Adleman)和ECC(Elliptic Curve Cryptography)。
下面是使用Go语言实现RSA非对称加密解密的示例代码:
package main import ( "crypto/rand" "crypto/rsa" "crypto/x509" "encoding/pem" "fmt" ) func encryptRSA(plainText []byte, publicKey *rsa.PublicKey) ([]byte, error) { encryptedText, err := rsa.EncryptPKCS1v15(rand.Reader, publicKey, plainText) if err != nil { return nil, err } return encryptedText, nil } func decryptRSA(encryptedText []byte, privateKey *rsa.PrivateKey) ([]byte, error) { decryptedText, err := rsa.DecryptPKCS1v15(rand.Reader, privateKey, encryptedText) if err != nil { return nil, err } return decryptedText, nil } func main() { privateKey, err := rsa.GenerateKey(rand.Reader, 2048) if err != nil { fmt.Println("Error generating RSA key pair:", err) return } publicKey := &privateKey.PublicKey plainText := []byte("Hello, World!") encryptedText, err := encryptRSA(plainText, publicKey) if err != nil { fmt.Println("Error encrypting with RSA:", err) return } fmt.Println("Encrypted text:", encryptedText) decryptedText, err := decryptRSA(encryptedText, privateKey) if err != nil { fmt.Println("Error decrypting with RSA:", err) return } fmt.Println("Decrypted text:", decryptedText) }
哈希算法
哈希算法是一种将任意长度的数据转换为固定长度摘要(哈希值)的算法。Go语言中支持的常用哈希算法有MD5和SHA(Secure Hash Algorithm)系列。
下面是使用Go语言实现MD5哈希算法和SHA-256哈希算法的示例代码:
package main import ( "crypto/md5" "crypto/sha256" "encoding/hex" "fmt" ) func md5Hash(data []byte) []byte { hash := md5.Sum(data) return hash[:] } func sha256Hash(data []byte) []byte { hash := sha256.Sum256(data) return hash[:] } func main() { data := []byte("Hello, World!") md5Hash := md5Hash(data) fmt.Println("MD5 hash:", hex.EncodeToString(md5Hash)) sha256Hash := sha256Hash(data) fmt.Println("SHA-256 hash:", hex.EncodeToString(sha256Hash)) }
通过上述的示例代码,我们可以看到在Go语言中实现加密解密算法是非常简单和高效的。对于更加复杂的情况,如加密解密大文件或者使用更高级的加密算法,Go语言的标准库中还提供了更多的相关函数和类,供开发者使用和扩展。